I want to call a secure Rest API using Javascript but its giving error.
the below code i am trying:
var request = new XMLHttpRequest();
var url = "http: // 10.10.50.10:8081/rsgateway/data/json/Number+9171246241";
var method = "GET";
request.open(method, url);
request.setRequestHeader("Access-Control-Allow-Headers", "*");
request.setRequestHeader("Access-Control-Allow-Credentials", "true");
request.setRequestHeader("Content-type", "application/json; charset=UTF-8");
request.setRequestHeader("Authorization", "Basic <test:Passw0rd@321 as Base64 encoded> ");
request.setRequestHeader("Access-Control-Allow-Origin", "*");
request.onreadystatechange = function () {
if (request.readyState ==4 && request.status ==200) {
var response = JSON.parse(this.response);
var sessionId = response.sessionId;
//Use this sessionId in all other calls
}
}
// Send request
console.log("OK");
request.send();
I am getting below errors in browser console:
XHROPTIONS
http :// 10.10.50.10:8081/rsgateway/data/json/Number+9171246241
CORS Missing Allow Origin
..........................................................................................................................................................
Cross-Origin Request Blocked: The Same Origin Policy disallows reading the remote resource at http://10.10.50.10:8081/rsgateway/data/json/Number+9171246241. (Reason: CORS header ‘Access-Control-Allow-Origin’ missing). Status code: 401.
while i try to call the api using basic auth with the postman and using test user its working fine.
please help me to fix it.
thanks,
What are the API headers?
these are the required header parameter.
http_method);
"Content-Type", "application/json; charset=UTF-8");
"Accept", "application/json");
setDoInput(true);
You are aware, that everyone can read your API credentials when you call the API from the client? This is normally a absolutely no go!
Are you sure the API is designed to accept requests from outside? Normally you get an CORS error if you try to fetch data from a server which only allow access from his own server or special whitelisted servers.
its an on premise service its ok, just i want to fix my JavaScript application to be able to call the api. if someone help/guide me, where i am doing wrong which i can not call successfully the api.
Yes, I am sure because i already have integrated with a Java client application which is working fine
Take a look at the browsers developer tools and show us the complete headers send to the API. Maybe the origin is missing
One more point, When i call the api from postman from the same VM which i am trying the javascript is working fine.
So you can easily compare the send headers from the postman console with the send headers in the browsers development tool and you will see the differentce
the only difference i am seeing on postman console is authorization.

Maybe i am using different/incorrect encoding of credentials.
But i am not sure which encoded method will be the correct one
this one is from browser console which is not working.
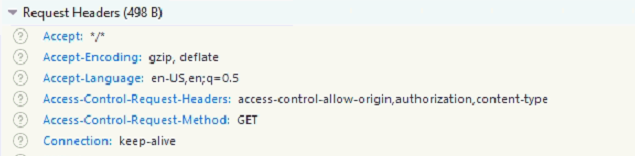
My test API have this settings
w.Header().Set(Access-Control-Allow-Methods, GET,HEAD,OPTIONS,POST,PUT,CREATE,DELETE)
w.Header().Set(Access-Control-Allow-Origin, *)
w.Header().Set(Access-Control-Allow-Headers, *)
w.Header().Set(Content-Type, application/json)
This is not ideal settings, but a starting point for further investigating.
after bringing some changes, i am getting these error in browser console.
Cross-Origin Request Blocked: The Same Origin Policy disallows reading the remote resource at http://10.10.50.10:8081/rsgateway/data/json/Number+9171246241. (Reason: CORS header ‘Access-Control-Allow-Origin’ missing). Status code: 401
Cross-Origin Request Blocked: The Same Origin Policy disallows reading the remote resource at http://10.10.50.10:8081/rsgateway/data/json/Number+9171246241. (Reason: CORS request did not succeed). Status code: (null).
I guess that this error is that the API server does not trust your requesting client.
Can you manage the API or is it a “foreign” API?
If it is a foreign API, you must obey their rules and set the request headers accordingly.
If you can manage the API, you must allow all incoming request to the API
Set(Access-Control-Allow-Origin, *) (* = all)
Note that this may not be recommended in production.
As i can see, postman is using client’s real ip address in request but my JavaScript is using 127.0.0.1 loopback ip address in request for Origin address. How can i hardcode Access-Control-Allow-Origin
to the client’s real ip address ?
As sibertius has pointed out, this is a problem with the response coming out of the server, not what you’re sending to it. That’s the point of CORS. It isnt up to the requesting client what CORS headers are sent, its up to the server.
Postman ignores CORS because it’s a dev tool. Your browser doesnt, because it’s meant for production use.